Neon Numbers are numbers that are the same as the sum of all digits of its squared number.
e.g: x2 = a+b+c…+n where (a,b,c) are all digits of x2.
For example, 9 is a neon number. Because
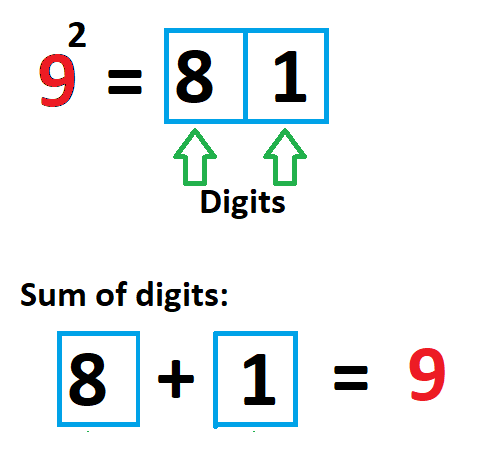
While 4 isn’t a neon number because of 42 = 16.And the sum of its digits is 1 + 6 = 7.And 7 not equals 4.
C/C++ code to check neon number
#include<iostream> using namespace std; int main(){ int inputNumber,square,rem = 0; cin >> inputNumber; square = inputNumber * inputNumber; int sum = 0; while(square!=0){ rem = square % 10; sum += rem; square /= 10; } if(inputNumber == sum ) cout << inputNumber << " is a neon number." << endl; else cout << inputNumber << " is not a neon number." << endl; }
So, you can see that first, we squared inputNumber and then put that in int variable square. Then we used the algorithm of the sum of digits of a number. Now if the sum is the same then the number will be a neon number or else not.
Program to check neon number in a given range in C/C++
Checking neon numbers in a specific range is easy. We just have to use a nested loop and then call the above snippet of code we used to check neon numbers. The only change in the above code will be the inputNumber will be the index of the first loop.
#include<iostream> using namespace std; int main() { int a,b,inputNumber,square; cin >> a >> b; for(a; a<=b; a++) { square = a * a; int sum = 0,rem = 0; while(square!=0) { rem = square % 10; sum += rem; square /= 10; } if(a == sum ) cout << a << " " << endl; } }